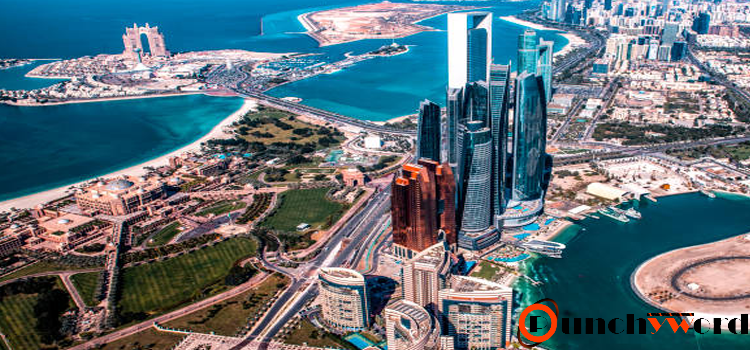
Laravel 7 Image uploading with validation complete example step by step procedure.
Today, I will show you how to create a simple image upload in Laravel 7. I write an article step by step about image upload in the Laravel 7 version with image validation.
I added image upload validation like image, mimes like jpeg, png, gif, and max file upload, So you can easily understand and use this code in your project.
We will create two routes one for GET method and another for the POSTmethod. We created a simple form with file input. You can select an image and then it will upload in the “images” directory of the public folder.
If you have an already installed Laravel project then you can simply skip the steps and just add the controller code function where we code for image uploading in Laravel.
Step 1: Install Laravel 7 App
First of all, we need to get a fresh Laravel 7 version application using bellow command because we are going from scratch, so open your terminal OR command prompt and run bellow command:
composer create-project –prefer-dist laravel/laravel blog
Step 2: Create Routes
In the next step, we have created two routes in the web.php file. One route for generate form and another for post method so let’s simply create both routes as bellow listed:
Folder: routes/web.php
Route::get('image-upload', 'ImageUploadController@imageUpload')->name('image.upload'); Route::post('image-upload', 'ImageUploadController@imageUploadPost')->name('image.upload.post');
Step 3: Create Controller
In the third step, we will have to create a new ImageUploadController and we have written two methods imageUpload() and imageUploadPost(). So one method will handle get method another one for post. So let’s add code.
app/Http/Controllers/ImageUploadController.php
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; class ImageUploadController extends Controller { /** * Display a listing of the resource. * * @return \Illuminate\Http\Response */ public function imageUpload() { return view('imageUpload'); } /** * Display a listing of the resource. * * @return \Illuminate\Http\Response */ public function imageUploadPost(Request $request) { request()->validate([ 'image' => 'required|image|mimes:jpeg,png,jpg,gif,svg|max:2048', ]); $rules = ['image' => 'required|image|mimes:jpeg,png,jpg,gif,svg|max:2048']; $request->validate($rules); $imageName = time().'.'.$request->file('image')->getClientOriginalExtension(); $request->file('image')->move(public_path('images'), $imageName); return back() ->with('success','You have successfully upload image.') ->with('image',$imageName); } }
Step 3: Create Blade File
In the last step, we need to create an imageUpload.blade.php file and in this file, we will create a form with a file input button. So copy bellow and put on that file.
resources/views/imageUpload.blade.php
<!DOCTYPE html> <html> <head> <title>Laravel 7 image upload example - HDTuto.com</title> <link rel="stylesheet" href="http://getbootstrap.com/dist/css/bootstrap.css"> </head> <body> <div class="container"> <div class="panel panel-primary"> <div class="panel-heading"><h2>Laravel 5.7 image upload example - HDTuto.com</h2></div> <div class="panel-body"> @if ($message = Session::get('success')) <div class="alert alert-success alert-block"> <button type="button" class="close" data-dismiss="alert">×</button> <strong>{{ $message }}</strong> </div> <img src="images/{{ Session::get('image') }}"> @endif @if (count($errors) > 0) <div class="alert alert-danger"> <strong>Whoops!</strong> There were some problems with your input. <ul> @foreach ($errors->all() as $error) <li>{{ $error }}</li> @endforeach </ul> </div> @endif <form action="{{ route('image.upload.post') }}" method="POST" enctype="multipart/form-data"> @csrf <div class="row"> <div class="col-md-6"> <input type="file" name="image" class="form-control"> </div> <div class="col-md-6"> <button type="submit" class="btn btn-success">Upload</button> </div> </div> </form> </div> </div> </div> </body> </html>
Step 4: Create “images” Directory
In the last step, we need to create new directory “images” with full permission, so let’s create a new folder in the public folder.
If you found any difficulties or issues you can comment to us. We will respond to you as soon as possible. and you can explore more on laravel official documentation.
Thanks