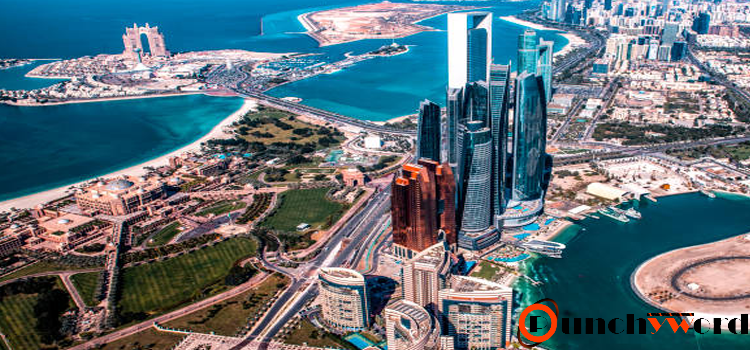
Convert HTML to PDF in Laravel using the domPDF library will give you a very simple way to convert HTML to PDF file and give to download that pdf step by step. In this tutorial, we use laravel-dompdf package barryvdh to convert HTML to pdf in laravel and this package also provides to download function.
Recently for a custom Laravel based Ecommerce project (which I am developing for a client company), I needed to export the order in the form of an invoice into pdf file where I use dynamic PDF generation feature for the customer’s order invoices so they could easily print the order invoices on pdf. After searching for some hours I found a very nice DOMPDF Wrapper for Laravel at GitHub, laravel-dompdf.
The Step by Step procedure is written you can follow all the steps properly. We assume you have already set up a Laravel project.
1. Package Installation
Now let’s move to our project directory where you want to install the package.
like: D:\xampp\htdocs\projectname
composer require barryvdh/laravel-dompdf
You need to wait for the installation of the package until you see the cursor starts blinking again at the end of the command interface.
2: Add service provider
Now open the config/app.php file
Add the following line to ‘providers’ array:
'providers' => [ .... Barryvdh\DomPDF\ServiceProvider::class, ],
Now add these lines to ‘aliases’:
'aliases' => [ .... 'PDF' => Barryvdh\DomPDF\Facade::class, ]
3. Update routes/web.php
Open the routes/web.php file and add the following route.
Route::get(order/print-pdf,'PdfController@orderPrintPDF')->name('order.print-pdf');
In the above, you see I have a function in orderPrintPDF() in PdfController that will handle the PDF generation. Now add that route to an anchor or button you want to use for PDF generation.
<a href="{{route('order.printpdf')}}">Print PDF</a>
4: Create Controller
Create a file in app/Http/Controllers/PdfController.php
add the given code.
<?php // Our Controller namespace App\Http\Controllers; use Illuminate\Http\Request; // This is important to add here. use PDF; class PdfController extends Controller { public function orderPrintPDF(Request $request) { // This $data array will be passed to our PDF blade $data = [ 'title' => 'First PDF for Medium', 'heading' => 'Hello from 99Points.info', 'content' => 'Lorem Ipsum is simply dummy text of the printing and typesetting industry.' ]; $pdf = PDF::loadView('pdf_view', $data); return $pdf->download('customorder.pdf'); } }
You can use the above function orderPrintPDF() into any of your existing controllers or you can create a new one with any other name (I am using PdfController as an example in this post). But make sure if you have a different controller name then you should update the above route lines (Step 3) accordingly.
5. Create Blade View
A blade view is a file that will be rendered to our final PDF. To apply formatting on a PDF file you need to add inline CSS to the elements and to make the elements fit the PDF use HTML tables instead of Div, Span, or other markups tags.
Don’t forget to give the table 100% width if you want to create a PDF with edge to edge layout.
Create a view pdf_view.blade.php in \resources\views\
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <title>{{ $title }}</title> </head> <body> <h1>{{ $heading}}</h1> <table width="100%" style="width:100%" border="0"> <tr> <td> </td> <td> </td> <td> </td> </tr> <tr> <td> </td> <td> </td> <td> </td> </tr> </table> </body> </body> </html>
I hope this example of HTML to pdf in laravel has made your work easier.
You can also check our Laravel Image Uploading with validation tutorials.