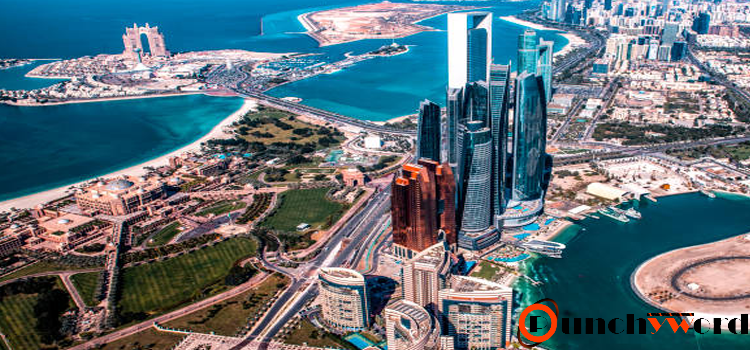
Send a push notification using firebase-admin in nodejs is very easy and common firebase announces a new package name firebase-admin you can download it from npm package repository.
Firebase provides the tools and infrastructure you need to develop your app, enhance your user base. The Firebase Admin Node.js SDK enables access to Firebase services from privileged environments (such as servers or cloud) in Node.js.
I am assuming you have set up the nodejs project on your machine, so you can install it from npm using your command-line interface.
Note: if you didn’t setup the firebase account then you can not process further, so you need to setup the firebase console account first then follow the below steps, Or you can click here to how to setup the project on firebase console guide:
You can also use the official firebase documentation from here.
Installation
$ npm install --save firebase-admin
var admin = require('firebase-admin');
var serviceAccount = “path of your configuration file .json”;
if (!admin.apps.length) {
admin.initializeApp({
credential: admin.credential.cert(serviceAccount),
databaseURL: {put here your firebase database url}
});
}
To use the module in your application, require it from any JavaScript file.
Service Account: is the .json file that is downloaded from a firebase project like this.
testfile-6c511-firebase-adminsdk-ww9ak-fe432d870e.json
database URL: firebase database URL which you want to connect like this “https://testdb-6c511.firebaseio.com”.
Note sendToDevice() method used for sending push to a single token if you want to send a push to multiple tokens you can use sendMulticast() method.
There are multiple methods available for sending push notifications using firebase-admin.
Using the Firebase Admin SDK or FCM app server protocols, you can build message requests and send them to these types of targets:
- Topic name
- Condition
- Device registration token
- Device group name (legacy protocols and Firebase Admin SDK for Node.js only)
You can send messages with a notification payload made up of predefined fields, a data payload of your own user-defined fields, or a message containing both types of payload. See Message types for more information or you can use this official link where all methods are available.
Complete code:
var admin = require('firebase-admin');
var serviceAccount= "test-firebase-adminsdk-ww9ak-fe432d870e.json";
if (!admin.apps.length) {
admin.initializeApp({
credential: admin.credential.cert(serviceAccount),
databaseURL: "https://test.firebaseio.com"
});
}
var token = "FCM DEVICE TOKEN Here";
var message = {
notification: {
title: "I need help.",
body: "Are you available for my help",
sound: "default"
},
data: {
title: "I need help.",
body: "Are you available for my help",
Emergency_category: "Emergency",
}
}
admin.messaging().sendToDevice(token, message).catch(console.error);
This is a complete example you can use in your project, if you found any issues you can comment here.