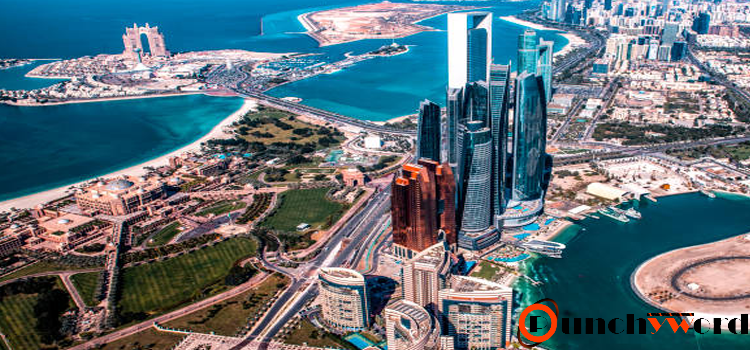
Stripe integration in Laravel is very easy you can integrate stripe in Laravel using some steps mentioned below.
We are supposed you have already set up laravel project and working properly and you have a good knowledge of laravel terms.
Establish stripe-php Package
This is a mandatory step of this tutorial, and you must have the composer installed on your development machine.
First, we need to install and configure the stripe-php plugin for creating stress-free payments in laravel.
We have to use this command in our command-line terminal for installing stripe-php.
>> composer require stripe/stripe-php
Configure Stripe Public & Secret API Keys
Stripe Public & Secret API Keys help us to create the connection between laravel and Stripe payment gateway.
We have to keep the Stripe Publishable and Secret key inside our .env file and make it secure. As we register the Stripe API keys in the env file, an agreement will be made between them.
- Now it’s time to create your account on stripe if it does not already exist go to the Stripe website and create your development account.
- Next, Get the Public and Secret API key from your development account.
- To restrict from making the real transaction, operate with a test account.
Put your stripe test key and secrete key in .env file and clear the laravel cache using the command
>> php artisan config:cache
STRIPE_KEY=pk_test_xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx
STRIPE_SECRET=sk_test_ xxxxxxxxxxxxxxxxxxxxxxxxxx
Now there are basically two ways to collect stripe payment in Laravel:
- Make a payment from the mobile application using REST API
- Make a payment from a web application
Make a payment from the mobile application using REST API
If you are using Laravel as a backend service REST API and make a payment through stripe payment gateway then mobile application developer integrate the Stripe SDK in the mobile app and who will generate the “nonce”. You just need two-parameter from mobile app developer in the payment API service:
- amount
- nonce
The mobile app developer will send these two parameters in your developed API for payment.
Let’s create a controller StripController.php and make a function makePayment(Request $request){} in StiperController.php and make a route in api.php
<?php
use App\Http\Controllers\StripeController;
use Illuminate\Support\Facades\Route;
Route::post('make-payment',[StripeController::class,'makePayment']);
This is an api.php file in which we create a post route make-payment which will be used by the mobile app developers and send two parameters in this service amount and nonce and then StripeController handles this request and sends the proper response in JSON format.
StripeController.php
<?php
namespace App\Http\Controllers;
use Exception;
use Illuminate\Http\Request;
class StripeController extends Controller
{
/**handling payment with POST API*/
public function makePayment(Request $request)
{
try{
/* Instantiate a Stripe Gateway either like this */
$stripe = new \Stripe\StripeClient(env('STRIPE_SECRET'));
$charge = $stripe->charges->create([
'card' => $request->nonce,
'currency' => 'USD',
'amount' => ($request->amount * 100),
'description' => "New Payment Received from mobile app",
'metadata' => [
"order_id" => 1111,
"others" => "You can add anything metadata"
]
]);
if($charge->status == 'succeeded') {
$data = ['transaction_id' => $charge->id];
return ['success'=>1,'message'=>'Transaction Success','data'=>$data];
}else{
return ['success'=>0,'message'=>'Card not charge, Please try again later','data'=>[]];
}
}catch(Exception $e){
return ['success'=>0,'message'=>"Error Processing Transaction",'data'=>[]];
}
}
}
Make a payment from web application
If you are creating a web application and want to make a payment using payment form view in laravel then you have to follow all the below steps completely.
We are supposed you have set up laravel project and stripe integration successfully.
- Create Two routes in web.php file.
<?php
use App\Http\Controllers\StripeController;
use Illuminate\Support\Facades\Route;
Route::get('stripe-payment,[StripeController::class,"stripePaymentForm"]);
Route::post('stripe-payment,[StripeController::class,"makeStripePayment"]);
2. Create two functions in StripeController.php
<?php
namespace App\Http\Controllers;
use Exception;
use Illuminate\Http\Request;
class StripeController extends Controller
{
public function stripePaymentForm(Request $request){
return view('payment-form');
}
public function makeStripePayment(Request $request)
{
$stripe = new \Stripe\StripeClient(env('STRIPE_SECRET'));
$charge = $stripe->charges->create([
'card' => $request->nonce,
'currency' => 'USD',
'amount' => ($request->amount * 100),
'description' => "New Payment Received from mobile app",
]);
Session::flash('success', 'Payment has been successfully processed.');
return back();
}
}
3. View Stipe Form and Validation
In this final step, we will create the form that accepts the card details along with some validation rules to validate the card information.
Create a payment-form.blade.php file, in here insert the following code altogether.
<!DOCTYPE html>
<html>
<head>
<title>Laravel 8 - Integrate Stripe Payment Gateway Example</title>
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/3.3.7/css/bootstrap.min.css" />
<script src="https://code.jquery.com/jquery-3.5.1.slim.min.js"></script>
<style type="text/css">
.container {
margin-top: 40px;
}
.panel-heading {
display: inline;
font-weight: bold;
}
.flex-table {
display: table;
}
.display-tr {
display: table-row;
}
.display-td {
display: table-cell;
vertical-align: middle;
width: 55%;
}
</style>
</head>
<body>
<div class="container">
<div class="row">
<div class="col-md-6 col-md-offset-3">
<div class="panel panel-default">
<div class="panel-heading">
<div class="row text-center">
<h3 class="panel-heading">Payment Details</h3>
</div>
</div>
<div class="panel-body">
@if (Session::has('success'))
<div class="alert alert-success text-center">
<a href="#" class="close" data-dismiss="alert" aria-label="close">×</a>
<p>{{ Session::get('success') }}</p>
</div>
@endif
<form role="form" action="{{ route('stripe.payment') }}" method="post" class="validation"
data-cc-on-file="false"
data-stripe-publishable-key="pk_test_51IEGBeAKkuZC4NpV1TgDIHam4nJPl5YiMGIo5jTeKV8yvPVjts6k6v9KEMJKC9D8tlLXvDCPFJXFx6dYIhQLzxeb00wXhzyiJ6"
id="payment-form">
@csrf
<div class='form-row row'>
<div class='col-xs-12 form-group required'>
<label class='control-label'>Name on Card</label> <input
class='form-control' size='4' type='text'>
</div>
</div>
<div class='form-row row'>
<div class='col-xs-12 form-group card required'>
<label class='control-label'>Card Number</label> <input
autocomplete='off' class='form-control card-num' size='20'
type='text'>
</div>
</div>
<div class='form-row row'>
<div class='col-xs-12 col-md-4 form-group cvc required'>
<label class='control-label'>CVC</label>
<input autocomplete='off' class='form-control card-cvc' placeholder='e.g 415' size='4'
type='text'>
</div>
<div class='col-xs-12 col-md-4 form-group expiration required'>
<label class='control-label'>Expiration Month</label> <input
class='form-control card-expiry-month' placeholder='MM' size='2'
type='text'>
</div>
<div class='col-xs-12 col-md-4 form-group expiration required'>
<label class='control-label'>Expiration Year</label> <input
class='form-control card-expiry-year' placeholder='YYYY' size='4'
type='text'>
</div>
</div>
<div class='form-row row'>
<div class='col-md-12 hide error form-group'>
<div class='alert-danger alert'>Fix the errors before you begin.</div>
</div>
</div>
<div class="row">
<div class="col-xs-12">
<input type="hidden" name="amount" value="100" />
<button class="btn btn-danger btn-lg btn-block" type="submit">Pay Now ($100)</button>
</div>
</div>
</form>
</div>
</div>
</div>
</div>
</div>
</body>
<script type="text/javascript" src="https://js.stripe.com/v2/"></script>
<script type="text/javascript">
$(function() {
var $form = $(".validation");
$('form.validation').bind('submit', function(e) {
var $form = $(".validation"),
inputVal = ['input[type=email]', 'input[type=password]',
'input[type=text]', 'input[type=file]',
'textarea'].join(', '),
$inputs = $form.find('.required').find(inputVal),
$errorStatus = $form.find('div.error'),
valid = true;
$errorStatus.addClass('hide');
$('.has-error').removeClass('has-error');
$inputs.each(function(i, el) {
var $input = $(el);
if ($input.val() === '') {
$input.parent().addClass('has-error');
$errorStatus.removeClass('hide');
e.preventDefault();
}
});
if (!$form.data('cc-on-file')) {
e.preventDefault();
Stripe.setPublishableKey($form.data('stripe-publishable-key'));
Stripe.createToken({
number: $('.card-num').val(),
cvc: $('.card-cvc').val(),
exp_month: $('.card-expiry-month').val(),
exp_year: $('.card-expiry-year').val()
}, stripeHandleResponse);
}
});
function stripeHandleResponse(status, response) {
if (response.error) {
$('.error')
.removeClass('hide')
.find('.alert')
.text(response.error.message);
} else {
var token = response['id'];
$form.find('input[type=text]').empty();
$form.append("<input type='hidden' name='nonce' value='" + token + "'/>");
$form.get(0).submit();
}
}
});
</script>
</html>
We completed all the above stipe integration in laravel hope you found it informative. If you have any confusion or get any problem related to stripe integration in laravel you can comment on it.